views
Introduction
Evaluating database scalability requirements in MEAN Stack Development projects is essential to ensure that applications can handle growth, maintain performance, and offer a seamless user experience. The MEAN stack, which consists of MongoDB, Express.js, Angular.js, and Node.js, is specifically well-suited for building scalable applications due to its architecture and components.
This detailed guide highlights the essential questions that you should consider when assessing scalability needs in MEAN Stack Development projects, along with strategies and best practices for effective implementation.
Let’s begin by understanding what MEAN stack development is?
Understanding the MEAN stack development
Before we talk about scalability considerations, it is important to understand the components of MEAN stack development:
- MongoDB: A NoSQL database offers flexibility and horizontal scalability, thus allowing you to handle large volumes of data efficiently.
- Express.js: A web application framework for Node.js which simplifies server-side development and facilitates communication between the front end and the back end.
- Angular.js: It is a front end framework which allows the development of dynamic single-page applications.
- Node.js: A server-side JavaScript runtime which supports high concurrency and is optimized for I/O heavy operations.
The combination of these components allows developers to build an efficient application that holds the capability to scale horizontally by adding more servers instead of upgrading existing hardware.
Now, quickly let us understand a few key questions that you should ask before evaluating scalability needs.
10 Questions to ask before Evaluating Scalability needs?
1. What are the current and expected user loads?
Understanding both present user loads and foresight growth would be extremely important. Consider:
- Current User Base: Number of active users of the application
- Projected Growth: What is the projected growth rate over the next year or two?
This information helps determine whether your existing infrastructure can handle future demands.
2. What type of data will be processed?
Different types of data have different scalability requirements.
- Volume: How much will be produced?
- Complexity: Will the data have complex relationships that require relational database features?
- Access Patterns: How often will the data be read or written?
MongoDB's document-based structure benefits unstructured data but can be more challenging to design in complex relationships.
3. What are the performance requirements?
Performance directly affects user experience. Some of the KPIs to consider in this include:
- Response Time: What is an acceptable response time for your application?
- Throughput: How many requests per second should your application handle?
- Latency: What is the tolerance level of latency during peak loads?
Defining these metrics helps in setting benchmarks for scalability efforts.
4. Will You Use Vertical or Horizontal Scaling?
Deciding what to scale vertically or horizontally is fundamental
- Vertical Scaling: It implies scaling up existing hardware like CPU, RAM. It does give an immediate improvement in performance but has drawbacks of cost and capacity.
- Horizontal Scaling: It's the addition of more servers to distribute loads.
This method is often much more cost-effective and fits in quite well with the distributed nature of MEAN stack applications.
Vertical Scaling
- Advantages: Immediate performance boost
- Disadvantages: Limited capacity; costly upgrades
Horizontal Scaling
- Advantages: Cost-effective; scalable; fault-tolerant
- Disadvantages: More complex architecture
5. What caching strategies will be used?
Caching can highly improve performance by minimizing database load:
- In-Memory Caching: Use Redis or Memcached to cache data that has to be accessed often.
- HTTP Caching: It's the caching at the HTTP level to reduce the server requests.
Consider how caching will be integrated into your application architecture.
6. How will you manage data growth?
As your application scales, managing data becomes very important:
Sharding: Spread data across several servers to enhance read/write performance.
Replication: Data is maintained across multiple servers for availability and fault tolerance.
Such strategies will help manage large datasets more effectively.
7. Monitoring Tools to Be Utilized
It is essential for identifying bottlenecks and ensuring optimal performance:
- Application Performance Monitoring (APM): Tools like New Relic or Datadog can provide insights into application health.
- Database Monitoring: Monitor query performance, slow queries, and resource usage in MongoDB.
It ensures that problems are addressed in advance before they impact users.
8. How Will You Manage Load Balancing?
Load balancing is important as it helps distribute traffic across servers equally.
Type of Load Balancer:
- Software-based solutions (e.g., Nginx)
- Hardware appliances
- Cloud-based services (e.g., AWS Elastic Load Balancing)
This balances loads so that no single server becomes a bottleneck, even in periods of heavy traffic.
9. What Is Your Deployment Strategy?
Deployment strategies affect scalability:
- Continuous Integration/Continuous Deployment (CI/CD): Automate deployment processes to facilitate quick updates without downtime.
- Containerization: Containerization-using Docker or Kubernetes for better management of microservices, which facilitates their easy scalability.
A well-defined deployment strategy enhances agility in responding to user demands.
10. Are there any security considerations?
Scalability does not compromise security:
- Data Encryption: encrypt sensitive data both at rest as well as in transit.
- Access Controls: Enforce strict access controls that prevent unauthorized access when your application scales.
Integrating security measures from the outset helps maintain user trust during growth phases.
Best Practices for Database Design in Scalable MEAN Stack Applications
Designing a database with scalability in mind is crucial for the long-term success of MEAN stack applications. Here are some best practices to consider when structuring your MongoDB database:
1. Schema Design and Data Modeling
Use Document-Oriented Design
MongoDB is a NoSQL database that uses a document-oriented data model. This allows for flexible schema designs. When designing your schema, consider the following:
- Embed Related Data: Instead of using multiple collections, embed related data within documents where appropriate. This reduces the need for complex joins and can improve read performance.
- Use References When Necessary: For large datasets or when data is frequently updated, use references to link documents instead of embedding. This approach helps maintain data integrity and reduces duplication.
Embedding Data
- Advantages: Faster reads; fewer queries needed
- Disadvantages: Can lead to large document sizes
Referencing Data
- Advantages: Maintains data integrity; reduces duplication
- Disadvantages: Requires additional queries for joins
2. Indexing Strategies
Proper indexing is critical for ensuring fast query performance:
- Create Indexes on Frequently Queried Fields: Identify fields that are commonly used in queries and create indexes on them to speed up search operations.
- Use Compound Indexes: When queries involve multiple fields, consider creating compound indexes that can optimize complex queries.
- Monitor Index Usage: Regularly review index usage statistics to identify unused indexes that can be removed, reducing overhead.
3. Data Partitioning and Sharding
As your application grows, managing large datasets becomes essential:
- Implement Sharding: MongoDB supports sharding, which distributes data across multiple servers. Choose an appropriate shard key that balances load evenly across shards while minimizing cross-shard queries.
- Monitor Shard Distribution: Regularly check the distribution of data across shards to ensure balanced load and optimal performance.
4. Optimize Read and Write Operations
Understanding the read/write patterns of your application can help optimize performance:
- Batch Writes: Instead of writing individual documents one at a time, group writes into batches to reduce the number of operations and improve throughput.
- Use Read Preferences: Configure read preferences based on your application’s needs. For instance, if eventual consistency is acceptable, consider reading from secondary replicas to distribute load.
5. Regular Maintenance and Refactoring
As your application evolves, so should your database design:
- Conduct Regular Reviews: Periodically review your database schema and indexing strategies to ensure they align with current application requirements.
- Refactor as Needed: Be prepared to refactor your database design based on changing data patterns or scaling needs. This may involve restructuring collections or adjusting indexes.
6. Documentation and Collaboration
Effective communication among team members is vital for maintaining a scalable database:
- Document Database Schema: Maintain clear documentation of your database schema, including relationships between collections and indexing strategies.
- Collaborate with Developers: Ensure that developers understand the implications of their code on the database design and performance. Foster collaboration between front-end and back-end teams to align goals.
Now, further let us understand the Strategies for effective Scalability in MEAN stack projects
What are the strategies for Effective strategies in MEAN Stack Projects?
To achieve an optimal scalability in MEAN stack application, consider to implement the following strategies:
Horizontal Scaling with Microservices
Transitioning to a microservice architecture would enable you to break down your application into smaller, manageable services which can scale independently. This approach enhances the flexibility and fault tolerance while simplifying the deployment processes.
Optimizing MongoDB performance
To ensure that MongoDB works efficiently under load:
- Implement sharding to distribute data across multiple nodes.
- Use replica sets for high availability and automatic failover.
- Monitor query performance regularly and optimize indexes whenever required.
Caching Mechanisms
Implement caching at various levels within the application architecture
- Database Caching: Cache frequent queries using Redis or Memcached.
- Application-Level Caching: Store results from expensive computations or API calls temporarily.
- Browser Caching: Leverage browser caching techniques to reduce server load during repeated visits.
Load Balancing Techniques
Use load balancing solution that distributes incoming traffic evenly across various servers:
- Use round-robin DNS or dedicated load balancers like HAProxy.
- Monitor server health continuously to redirect traffic away from unhealthy nodes.
- Configure auto-scaling groups in cloud environments to automatically adjust resources based on the demand.
Continuous Monitoring and Optimization
Frequently monitor application performance using APM tools:
- Identify bottlenecks in real time
- Analyze user behavior patterns to forecast traffic spikes.
- Optimize resource allocation based on the usage trends.
Now further let us understand how Can I help?
How Can Acquaint Softtech help?
As an official Laravel Partner and software development outsourcing business, Acquaint Softtech provides IT staff augmentation to reduce talent shortages in the organization's in-house development teams.
In addition, we have been specializing in MEAN stack and MERN stack development for the past 11 years. We have completed some really exciting projects from a variety of industries, including FinTech, Real Estate, EdTech, etc., by assisting them to hire MEAN stack developers or hire MERN stack developers, or by providing them with software development services that are outsourced.
To enable businesses to meet their demands at the most competitive pricing and hence deliver cost savings, recruiting rates to hire remote developers begin at a minimum of $15 per hour. If you’re looking for a cost-effective Laravel development solution, the cost of Laravel development varies based on project complexity, required expertise, and scope.
In addition to our previous growth in the United States and the United Kingdom, we have recently been extending our roots by growing our businesses in New Zealand. Our headquarters and official registration are in India.
Conclusion
MEAN stack projects become critical if proper analysis on database scalability needs are to be evaluated such that the application could be both scalable and growing effectively in the face of retaining their optimum performance. This means questions in development that seek the correct loading for what users require and which data kinds to satisfy certain levels of requirements of performances.
Another equally important aspect of successful database design is the inclusion of the best practices. Focusing much on schema design, indexing, sharding, and reads/writes will help ensure creation of a robust MongoDB architecture that supports both the demand of the present and future growth. Even regular maintenance and effective collaboration on the part of team members provide a good boost to ensure scalability and reliability.
This strategy will ensure that applications using MEAN Stack Development, mechanisms of caching, and techniques for load balancing are properly handled with increased traffic without compromising the user experience. Continuous monitoring and optimization are important in MEAN Stack Development to identify bottlenecks proactively and maintain high performance.
This strategy in the long run opens ways to fully utilize the MEAN stack from developers with the thought that how much they can balance between the demand for scalability, by using practical design and implementation techniques.
This would yield an application, which not only performs itself but also gains the satisfaction and trust of users as the application scales to the requirements of its users' needs and requirements. It will allow for building robust applications responsive to the changing digital environment to meet today's needs.
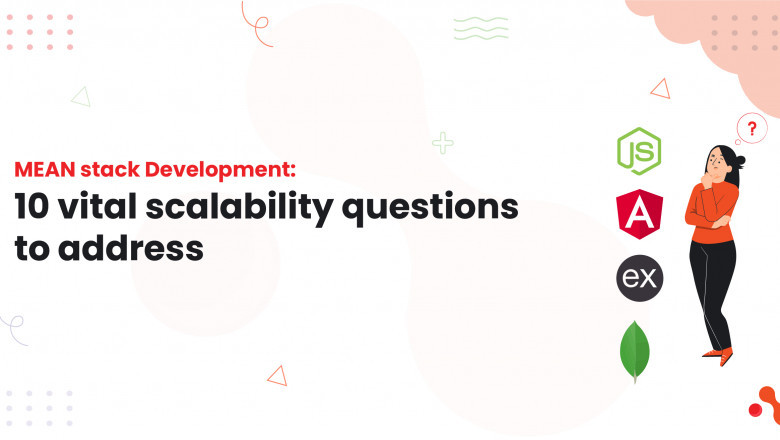

Comments
0 comment